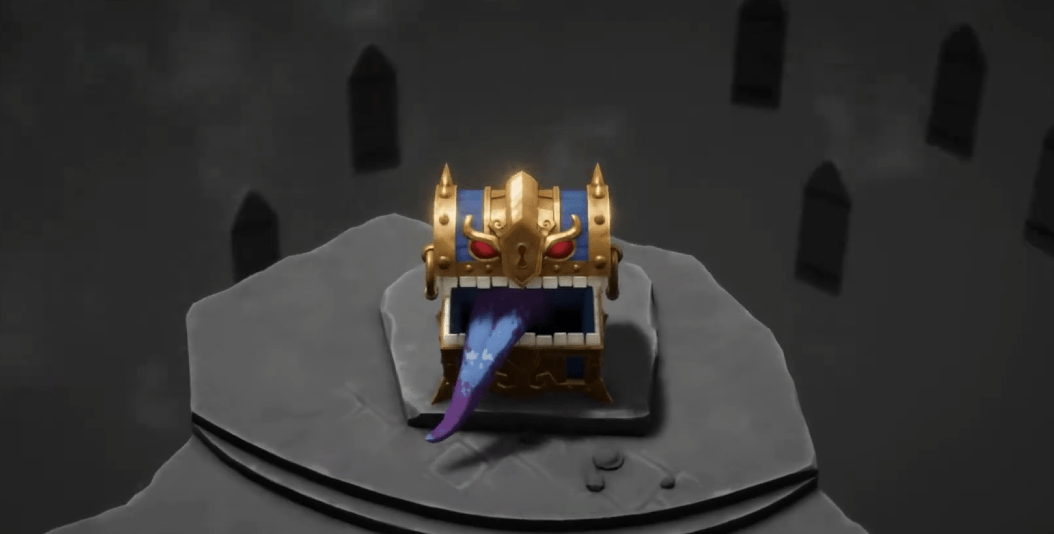
Mimic Game Devlog #1

You are The Mimic
Good Day! I’ve started development on a new game where you play as a mimic. Details are fuzzy, but I want to share some of my progress this first week.
For context, this game is being designed as a TURN-BASED and TILE-BASED traditional roguelike.
I can NOT be relied upon for weekly or even monthly updates. I expect future development logs to be irregular.
But first, please consider subscribing to my newsletter, either with the substack link below or with the RSS feed for this blog. I’m excited to share my game development journey with you.
Unrelated, I’ve been playing a lot of Death’s Door (where this image is from) and it’s a very fun game. Highly recommended.
Design Patterns and Foundation
I don’t have much in the way of gameplay or screenshots to share as I’ve been very focused on getting some passable foundations under this project.
Actors
Actors are all the little creatures, heroes, and such that act in the game, and it’s important to start strong with them. In Godot, we have a lot of opportunity for inheritance; I wanted to rely on that without going overboard.
The first draft looked something like this where each # comment represents a snippet of a new file.
# actor.gd
class_name Actor
# hero.gd
extends Actor
class_name Hero
# creature.gd
extends Actor
class_name Creature
I soon realized a potential flaw. Since I was making a game about being a Mimic… I want the mimic to eventually eat stuff. I want the hero to be able to eat food (re: items) AND creatures (re: actors). I may regret it later, but I functionally removed actors and replaced them with game objects. This way I can use inheritance for things like items and have them occasionally function the same way as an actor.
# game_object.gd
class_name GameObject
# hero.gd
extends GameObject
class_name Hero
# creature.gd
extends GameObject
class_name Creature
This should also give some flexibility for a specific use case I have in mind… other mimics! A Treasure Chest is a game object, but probably not an item or an actor. A Mimic (re: actor) can be a treasure chest + some additional functionality, which leads directly into…
Components
Inheritance IS NOT the answer to all life’s little problems, so I’m attempting to leverage components to gain some additional modularity with my game objects. My current system probably needs some optimization, but it looks a little like this.
# game_object.tscn
GameObject
→ Sprite2D
# hero.tscn
Hero
→ Sprite2D
→ MoveComponent
→ PlayerComponent
# creature.tscn
Creature
→ Sprite2D
→ MoveComponent
Game objects, heroes, and creatures all share a sprite for rendering. Creatures and heroes can move, thus they have a move component. The hero is controlled by the player, and so has a player component.
With a setup like this, I can conceivably have multiple actors controlled by the player. I can have actors controlled by the player that can’t move. I can grant any game object the ability to move independent from whatever else that game object is or does.
Like I said, I have a few kinks in this system to work through, mostly around finding the most efficient way to identify game objects with specific components.
Actions
Actions are something new that I’m trying to get a feel for. It’s an example of the Command pattern (I think) that will hopefully allow me to separate code that does things away from the game objects that do them. I think an example is required.
# action.gd
extends Resource
class_name Action
func perform() -> void:
pass
# move_action.gd
extends Action
class_name MoveAction
var game_object: GameObject
var position: Vector2
func perform() -> void:
game_object.position = position
The base Action class includes a perform function. The extended class MoveAction also includes the perform function, but also holds a reference to a game object and a position. When we ask our move component to move a game object, it returns a move action. We can store up a list of actions returned by game objects, then execute their perform functions in the correct order.
NOW…
I have some issues with this, and will probably refactor it later BUT it’s been helpful for me to work through some of those issues in my journey to learn and grow. Most likely I will shift to performing actions as they come to avoid any potential issues with freed game objects retaining a reference in a later action. It could be that this shouldn’t be it’s own thing at all and the move component should just handle the movement. I don’t know yet.
Unit Testing
I’ve never unit tested in Godot before, but I’m having success with GUT. By putting together some basic tests now, I’m finding it helpful to use them to ensure a function is working as expected in a more straightforward way? Previously I would create a lot of temporary objects or call functions in the _ready function of main just to have a quick way to test things out. Unit testing offers an opportunity to to shift that testing over to a dedicated space. I don’t plan to hit full test coverage or anything like (especially as I refactor stuff), but I’m hoping it will help me avoid at least a few bugs
Maps and Tiles
My next task is figure out a baseline for how to handle tiles and the map. Here is my initial draft (that will change).
# tile_type.gd
extends Resource
class_name TileType
var name: String
var walkable: bool
var sprite_atlas_coords: Vector2
# tile.gd
extends Resource
class_name Tile
var position: Vector2
var tile_type: TileType
# map.gd
extends Resource
class_name Map
var tiles: Array
Map.tiles is then instantiated as an Array of Array[Tile] where each tile position matches the position in the array (potentially redundantly) and has a tile type. The tile type then contains enough information for a semi-unrelated rendering node (TileMapLayer) to render it to the screen.
With some debug tiles and code, I managed to get a small Map to render along with a game object (skull), hero (chest), and creature (creature?).
The tileset I’m currently using is Oh No, More Goblins by MRMO Tarius. Please check it out, they are great. https://mrmotarius.itch.io/moregoblins
Anyway, that’s it. Thank you for reading. Please let me know if you have any comments or suggestions. I’d especially love to hear ways to make this project better. Please subscribe if you like.
nuzcraft